Näillä ohjeilla voit tehdä Android-tabletille (tai puhelimelle) meri- ja peruskartat, joiden käyttö ei vaadi verkkoyhteyttä. Valitsemasi kartat ladataan maksuttomista karttapalveluista tietokoneella ja siirretään tablettiin. Voit siis ladata vain ne alueet, joita oikeasti tulet käyttämään. Jos et halua ladata karttoja etukäteen, katso toinen ohje.
Karttaselaimena käytetään erinomaista OruxMaps-ohjelmistoa, joka on maksullinen.
Liikenneviraston karttapalvelusta ladattavat merikartat eivät sisällä saarien nimiä tai veneväyliä, mutta alla oleva asetustiedosto lataa ne Maanmittauslaitoksen rajapinnasta.
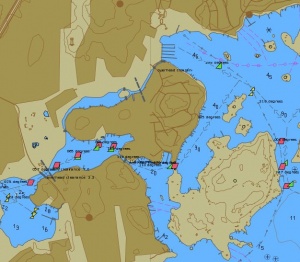
Jos resepti tuntii työläältä, kokeile vaikka Maastokarttoja.
A) Valmistelevat toimenpiteet
Kartat ladataan maksuttomalla MOBAC-ohjelmalla. Se on kirjoitettu karttatiedostojen lataukseen ja pakkaukseen eri ohjelmille.
- MOBAC tarvitsee Javan.
- Windows: Lataa Java. Se on maksuton.
- Linux: Tarvitset Javan. OpenJDK käy, esim. Ubuntussa asenna paketti “openjdk-7-jre”.
- Lataa MOBAC. Tuloksena on ZIP-tiedosto.
- Windows: Pura ZIP-tiedosto esim. työpöydälle.
- Linux: Pura ZIP-tiedosto esim. kotihakemistoosi.
- Tarvitset vielä meri- ja peruskarttojen määrittelytiedostot. Löydät ne tästä tiedostosta. Pura tiedostot MOBAC-ohjelman hakemistoon “mapsources”:
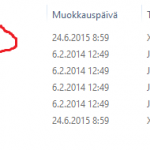
B) Valitse ja lataa kartat
MOBAC-ohjelman toimintaperiaate on yksinkertainen. Sen avulla voit tehdä atlaksia (karttakokoelmia), jotka sisältävät yhden tai useampia suorakaiteen muotoisia karttoja.
- Käynnistä MOBAC:
- Windows: Kaksoisnapsauta MOBAC-ohjelman hakemistosta löytyvää tiedostoa “Mobile Atlas Creator.exe”
- Linux: Aja “start.sh”, esim. terminaalissa “sh start.sh”.
- Anna ensimmäiselle atlakselle jokin kuvaava nimi tai esim. “Testi”. Valitse formaatiksi “OruxMaps Sqlite”.
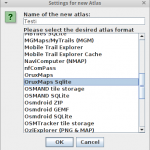
- Oletuksena sinulle näytetään karttaa “OpenStreetMap MapQuest” (ks. vasemman ylänurkan Map Source -valinta). Siirry tämän kartan avulla haluamallesi alueelle (esim. Suomenlahdelle).
- Liikuta karttaa pitämällä hiiren kakkosnappia alhaalla.
- Zoomaa hiiren rullalla tai näytön yläreunassa näkyvällä zoom-valitsijalla.
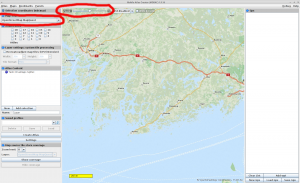
- Kun olet oikealla alueella ja zoom levelillä 10, valitse Map Source -valinnalla “Merikartta Liikennevirasto S57”.
- Rajaa haluamasi alueet (hiiren vasen näppäin), valitse zoom levelit ja valinta atlakseen (valinnan jälkeen vasemmalta Add selection).
- Valitut alueet (selection) vastaavat ikään kuin karttalehtiä, kun taas atlakset ovat kartastoja.
- Tabletin ohjelma osaa valita automaattisesti oikean karttalehden.
- Mitä isomman zoom levelin (vasen ylänurkka, 0-18) otat mukaan, sen enemmän tilaa kartta-aineisto vie (ja kartan tekeminen kestää). Kannattaa kokeilla jättää levelit 15-18 pois, niin säästyy tabletilta levytilaa.
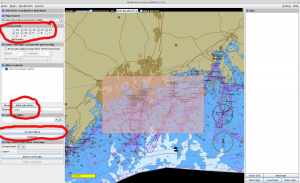
- Kun olet tyytyväinen atlakseen napsauta “Create atlas”. Se lataa valitsemasi kartat . Tämä kestää kauan, koska karttatiilet (kartan palaset) haetaan yksitellen palvelimelta. Mitä isompia zoom leveleitä olet valinnut selectioneihin, sen enemmän ladattavaa on.
- Valmis tavara tulee mobac-ohjelman hakemistoon “atlases”, jossa on alihakemisto kaikille tehdyille atlaksille.
C) Kartat Androidiin
Tässä vaiheessa olet tehnyt tietokoneella haluamasi kartat. Viimeisessä vaiheessa näytät kartat tabletissa.
- Lataa Androidille kartat näyttävä ohjelma OruxMaps.
- Käynnistä OruxMaps, jotta se tekee karttahakemistot tabletille. Voit sulkea OruxMapsin saman tien.
- Seuraavaksi voit siirtää valmiit kartat MOBAC-ohjelman hakemistosta “atlases” tabletille.
- Näet tabletin hakemiston seuraavasti: Asetukset (kolme pistettä näytön ylänurkassa) > Kaikki asetukset > Kartat > Kartat hakemisto: esim. “/storage/emulated/0/oruxmaps/mapfiles/”.
- Voit tehdä siirron esim. muistikortin tai Google Driven avulla. Kätevä apuväline tässä on Cheetah Mobilen File Manager.
- OruxMapsissa karttavalikko (karttaikoni) > Switch map > Offline > oma Atlaksesi jokin layer. Jos karttasi ei näy, paina yläreunan reload-painiketta.
D) OruxMapsin käyttö
OruxMapsissa on tärkeä säätää karttojen zoom-tasot oman päätelaitteen näytölle sopivaksi. Zoom-tasot näkyvät näytön oikeassa alanurkassa:
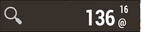
Zoom level on 16, joka on suurennettu digitaalisella zoomilla 136%:iin. Tässä näkyvät zoom levelit ovat samoja, jotka näkyvät MOBAC-karttoja tehdessä.
- Zoom leveliä voi vaihtaa nipistämällä karttaa kahdella sormella.
- Digital zoomia voi säätää äänenvoimakkuusnäppäimillä.
Pieni digital zoom auttaa, jotta karttamerkit näkyy sujuvasti. Zoom-asetuksia voi säätää: Asetukset (kolme pistettä näytön ylänurkassa) > Kaikki asetukset > Kartat > Zoom asetukset:
- Pyöritys ele: Ota tämä pois päältä, jos et halua kääntää karttoja.
- Äänenv. näppäimet: Valitse tämä, niin voit säätää digitaalisia zoomeja äänenvoimakkuuksilla.
- Viimeistele nipistys zoom: Valitse tämä, niin zoom level vaihtuu loogisesti.